Why Nue
Apps lighter than a React button
Nue is a standards-first framework that pushes HTML, CSS, JavaScript, and WASM to their limits. It helps you achieve more with significantly less code:
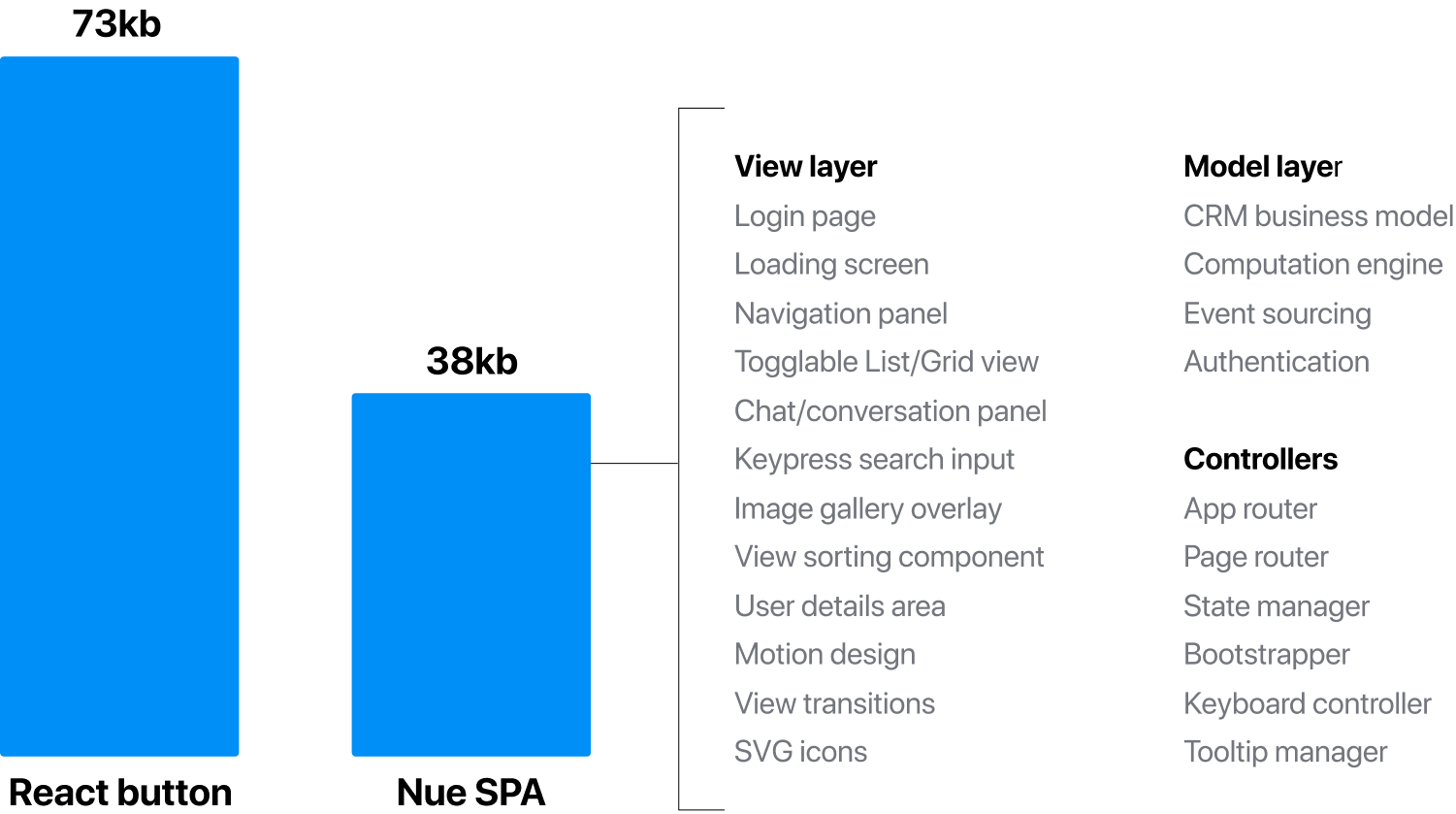
See benchmark and details here.
Extreme performance with Rust and WASM
The following demo uses Rust and Event Sourcing for instant keypress search over 150,000 — far beyond where JavaScript (and React) will crash with a stack overflow error:
Nue apps use a separated model layer to shatter the limits of traditional frameworks
Millisecond HMR and build speeds
Nue outperforms today’s frameworks across every key metric: bundle sizes, amount code needed, HMR speed, and build times.
Build times consistently run in milliseconds, even for large-scale apps, delivering orders of magnitude boosts over traditional tools. Here's how our demo is built and optimized for production:
What this means
For software architects
Nue brings back the good practices of software engineering: simple, testable functions, modular design, minimal dependencies, and a arcutecture-first approach. Build computation engines with the language of your choice (JavaScript, Rust, Go, or TypeScript) and reach new levels of performance and architectural clarity. Nue is for developers who wish to bulid on classic algorithms and data structures over React idioms.
For design engineers
Building a new theme shouldn’t require 40,000+ lines of TSX. Instead, you’ll craft a design system with modern CSS using @layers, variables, and calc(). You shift from React engineering to design engineering and start thinking about design topics like typographic scales and whitespace consistency.
For UX engineers
Imagine building entire applications with the same code footprint as a single React button, focusing solely on the user experience. Nue is the easiest way to build large-scale applications that push the web (and your skills) forward.
What’s next
Nue is an ambitious project that reshapes the entire frontend ecosystem:
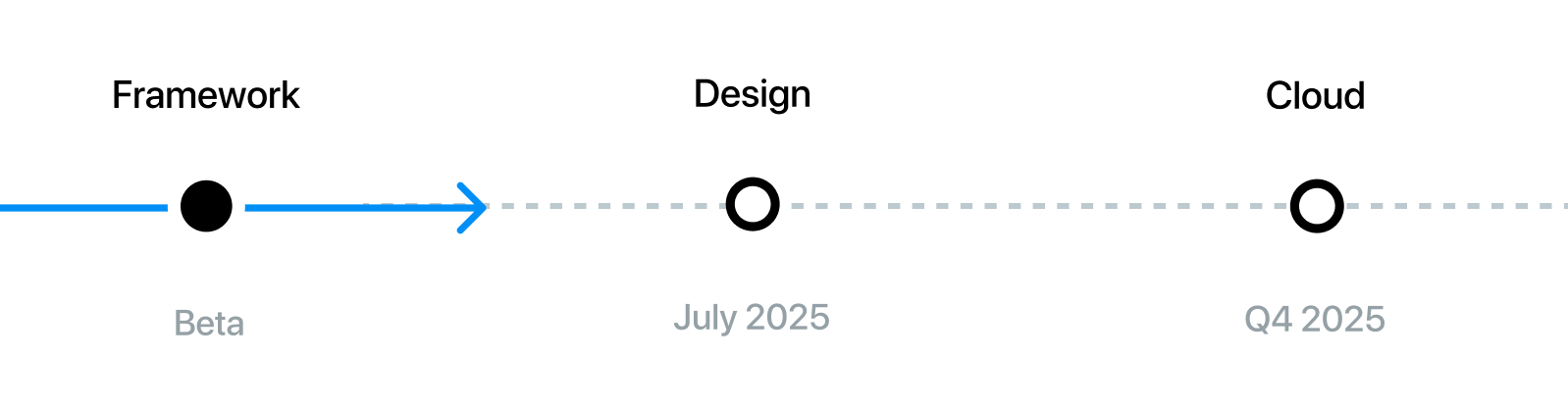
Find answers to common questions in our FAQ.
Please see how our vision unfolds.